Model Training Guide
A comprehensive step-by-step guide to training various types of machine learning models across different scenarios
What You'll Learn
Understanding model training fundamentals
Supervised vs. unsupervised training approaches
Handling different data types and distributions
Hyperparameter optimization strategies
Avoiding common training pitfalls
Evaluating model performance
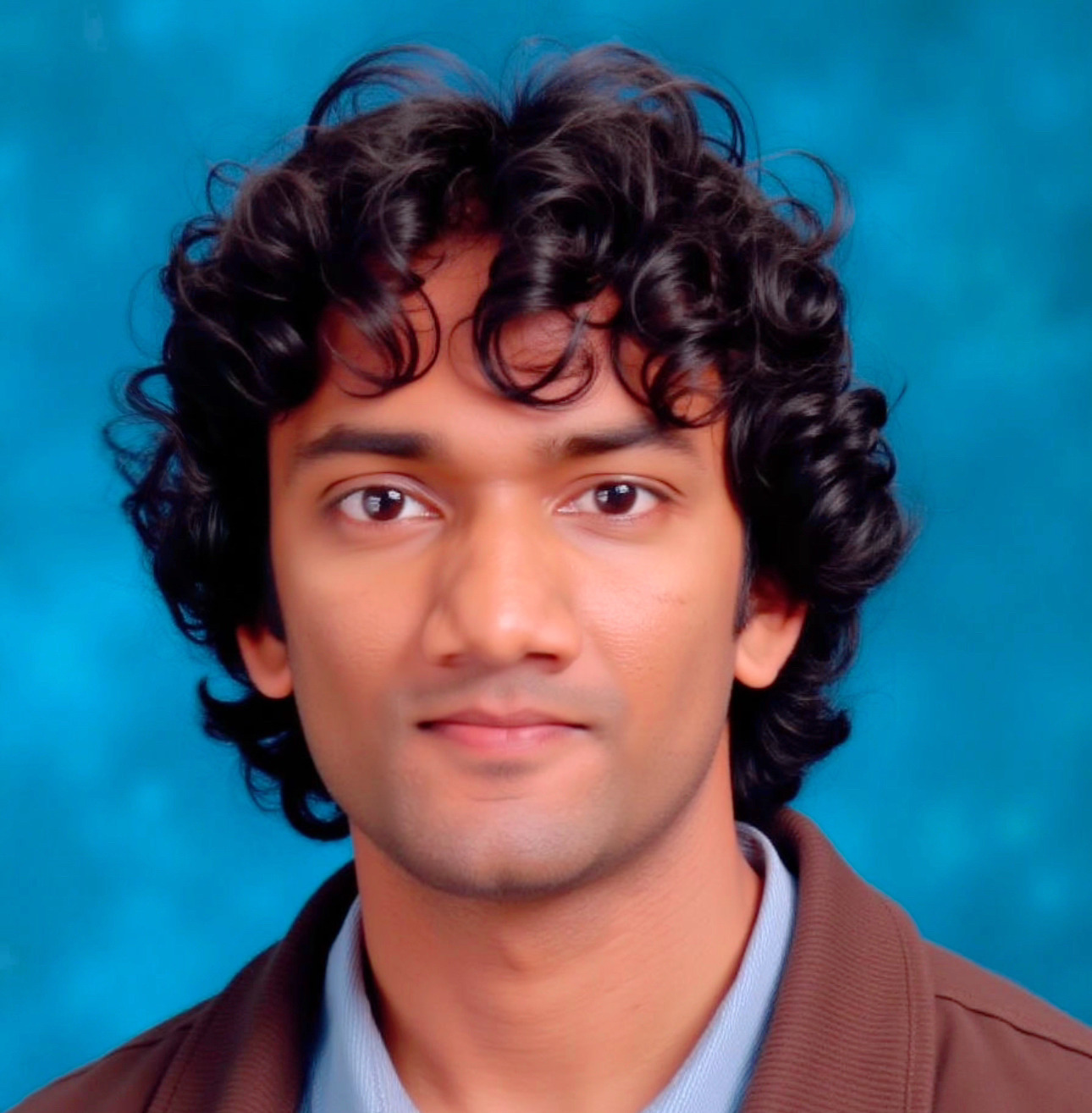
Nim Hewage
Co-founder & AI Strategy Consultant
Over 13 years of experience implementing AI solutions across Global Fortune 500 companies and startups. Specializes in enterprise-scale AI transformation, MLOps architecture, and AI governance frameworks.
View All TutorialsModel Training Scenarios
Tabular Data Classification
Training classification models on structured tabular data
Training Steps
- 1Data exploration and understanding class distribution
- 2Feature selection and engineering for tabular data
- 3Handling categorical variables and missing values
- 4Model selection: decision trees, random forests, and gradient boosting
- 5Cross-validation strategies for robust evaluation
- 6Hyperparameter tuning with grid search and Bayesian optimization
Recommended Tools
# Example code for training a classification model on tabular data import pandas as pd from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import classification_report # Load data data = pd.read_csv('customer_data.csv') X = data.drop('churn', axis=1) y = data['churn'] # Split data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train model model = RandomForestClassifier(n_estimators=100, max_depth=10) model.fit(X_train, y_train) # Evaluate predictions = model.predict(X_test) print(classification_report(y_test, predictions))
Best Practices
Always split your data into training, validation, and test sets
Ensure your validation strategy reflects the real-world use case
Start with simple models before moving to complex ones
Monitor for overfitting by comparing training and validation metrics
Use appropriate evaluation metrics for your specific problem
Document your experiments, including hyperparameters and results
Consider model interpretability alongside performance
Check for data leakage that could artificially inflate performance
Continue Your Learning Journey
Ready to apply what you've learned? Check out these related tutorials to deepen your understanding of model training and deployment.