Model Deployment
Guide to deploying machine learning models to production environments with DevOps and MLOps best practices
What You'll Learn
Understanding model deployment requirements
Containerization of ML models
CI/CD pipelines for ML workflows
Monitoring and observability strategies
Scaling and load balancing techniques
MLOps architecture and best practices
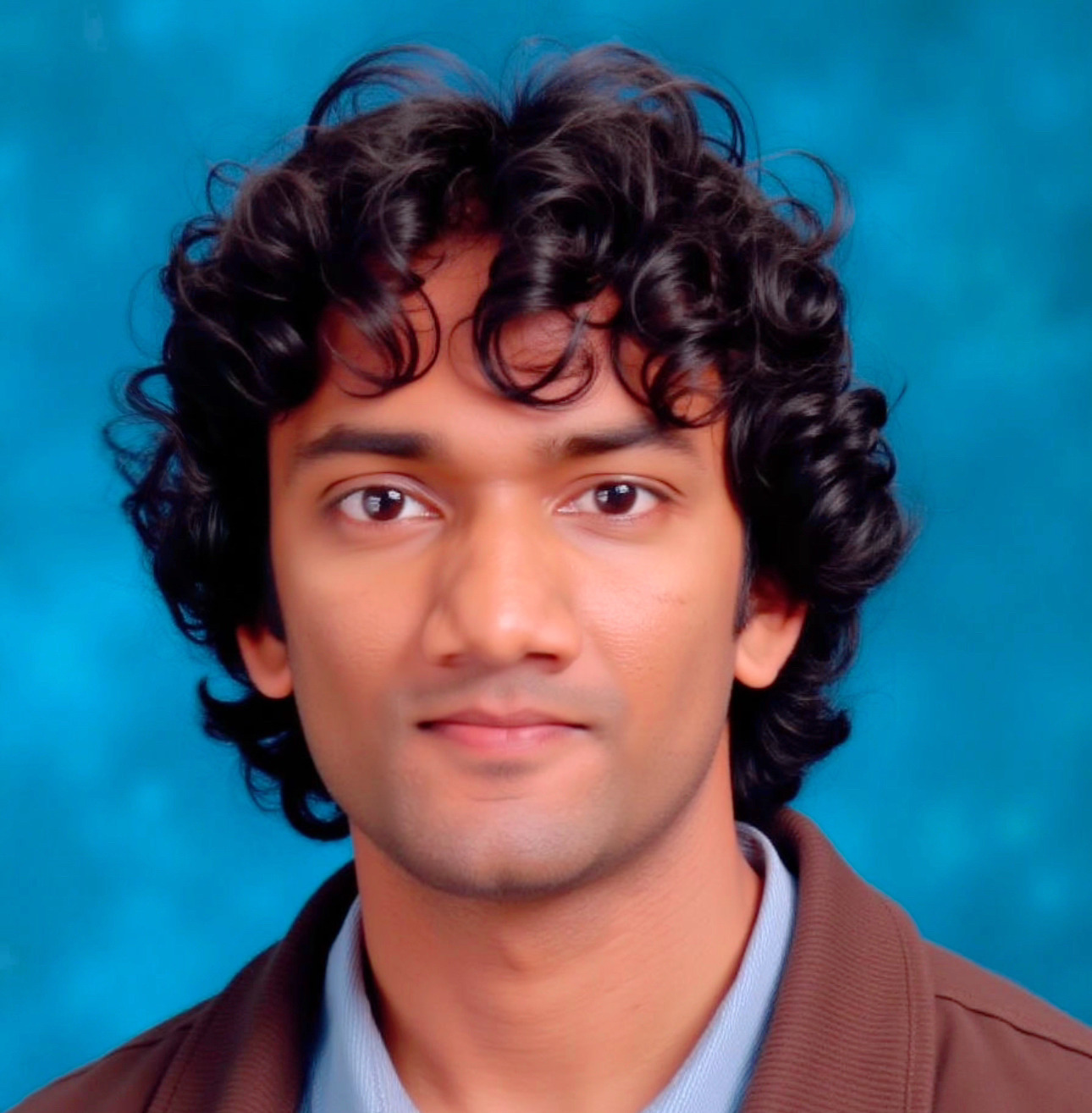
Nim Hewage
Co-founder & AI Strategy Consultant
Over 13 years of experience implementing AI solutions across Global Fortune 500 companies and startups. Specializes in enterprise-scale AI transformation, MLOps architecture, and AI governance frameworks.
View All TutorialsModel Deployment Approaches
API Deployment
Deploying machine learning models as RESTful APIs for applications to consume
Implementation Steps
- 1Package model and dependencies
- 2Create API endpoints to handle predictions
- 3Implement input validation and preprocessing
- 4Set up request/response serialization
- 5Configure authentication and rate limiting
- 6Deploy API with horizontal scaling capabilities
Recommended Tools
# Example of Flask API deployment for ML model import pickle import numpy as np from flask import Flask, request, jsonify app = Flask(__name__) # Load the pre-trained model with open('model.pkl', 'rb') as f: model = pickle.load(f) @app.route('/predict', methods=['POST']) def predict(): # Get data from request data = request.json # Validate input if not data or 'features' not in data: return jsonify({'error': 'No features provided'}), 400 # Preprocess input try: features = np.array(data['features']).reshape(1, -1) except Exception as e: return jsonify({'error': f'Invalid features format: {str(e)}'}), 400 # Make prediction try: prediction = model.predict(features).tolist() if hasattr(model, 'predict_proba'): probabilities = model.predict_proba(features).tolist() response = { 'prediction': prediction[0], 'probabilities': probabilities[0] } else: response = {'prediction': prediction[0]} return jsonify(response) except Exception as e: return jsonify({'error': f'Prediction error: {str(e)}'}), 500 # Health check endpoint @app.route('/health', methods=['GET']) def health(): return jsonify({'status': 'healthy'}) if __name__ == '__main__': app.run(host='0.0.0.0', port=8000)
MLOps Reference Architecture
A comprehensive architecture for ML model lifecycle management
Data Pipeline
Automated workflows for data collection, validation, and preprocessing
Key Elements
- Data ingestion services
- Data validation checks
- Feature engineering pipelines
- Data versioning and lineage tracking
- Feature store integration
Best Practices
Automate the entire model deployment pipeline
Implement comprehensive monitoring and observability
Version all model artifacts, data, and code
Design for scalability from the beginning
Ensure security at every step of the deployment process
Enable easy rollbacks to previous model versions
Document deployment architecture and procedures
Implement canary deployments for risk mitigation
Continue Your Learning Journey
Ready to apply these deployment techniques? Check out these related tutorials to enhance your DevOps and MLOps skills.