Data Preprocessing
Essential techniques for cleaning, transforming, and preparing data for machine learning models
What You'll Learn
Understanding data quality assessment
Handling missing and outlier data
Feature scaling and normalization
Categorical data encoding techniques
Feature selection and dimensionality reduction
Data augmentation strategies
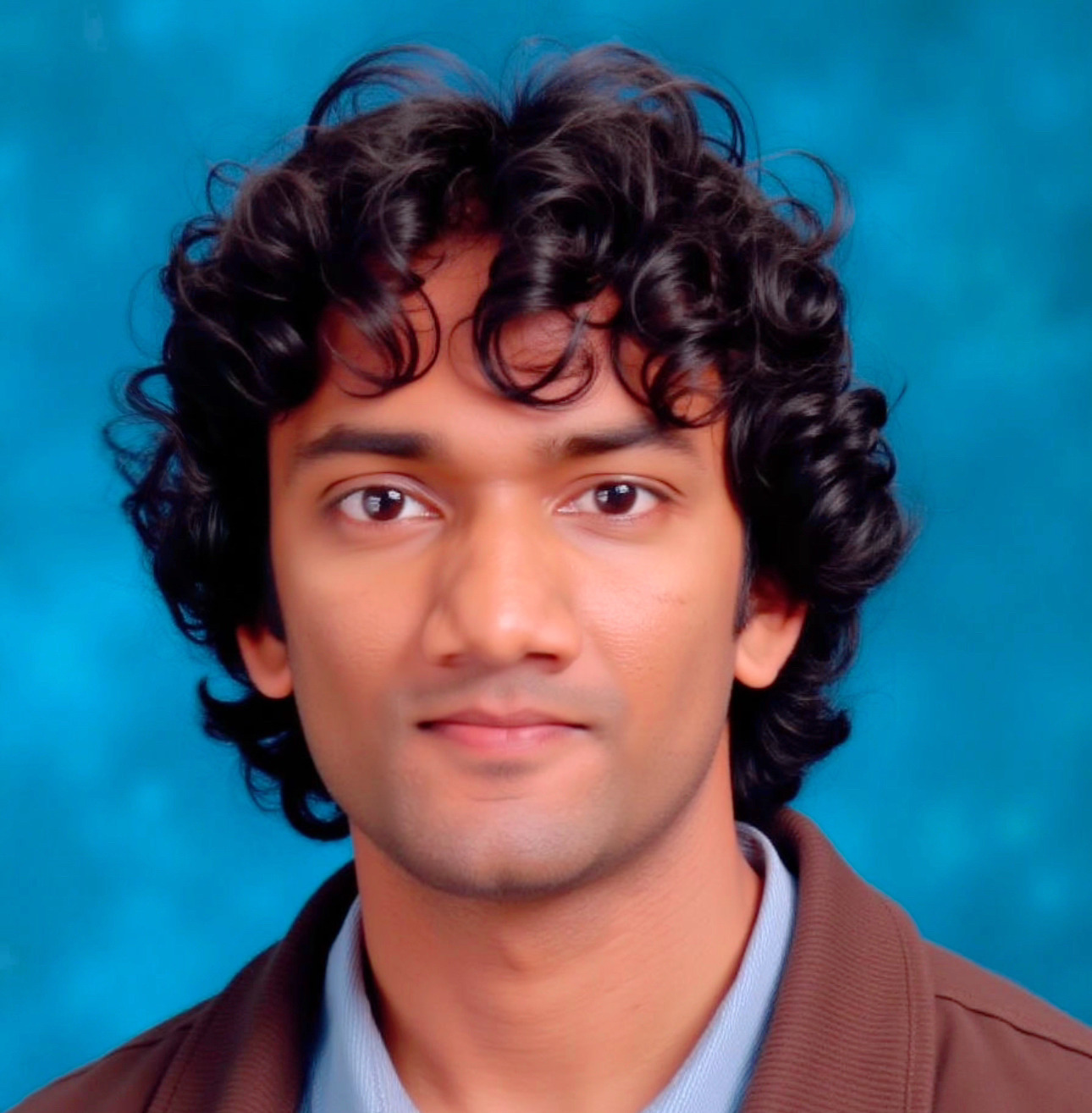
Nim Hewage
Co-founder & AI Strategy Consultant
Over 13 years of experience implementing AI solutions across Global Fortune 500 companies and startups. Specializes in enterprise-scale AI transformation, MLOps architecture, and AI governance frameworks.
View All TutorialsData Preprocessing Techniques
Data Cleaning
Identifying and handling missing values, outliers, and inconsistencies in your dataset
Implementation Steps
- 1Identify missing values and their patterns
- 2Choose appropriate missing value imputation techniques
- 3Detect and handle outliers with statistical methods
- 4Fix data inconsistencies and standardize formats
- 5Validate data types and ranges
- 6Document cleaning steps for reproducibility
Recommended Tools
# Example code for data cleaning import pandas as pd import numpy as np from sklearn.impute import SimpleImputer # Load data df = pd.read_csv('customer_data.csv') # Check for missing values print(f"Missing values per column:\n{df.isnull().sum()}") # Handle missing numerical values with median imputation num_imputer = SimpleImputer(strategy='median') numeric_cols = df.select_dtypes(include=['float64', 'int64']).columns df[numeric_cols] = num_imputer.fit_transform(df[numeric_cols]) # Handle missing categorical values with most frequent value cat_imputer = SimpleImputer(strategy='most_frequent') categorical_cols = df.select_dtypes(include=['object']).columns df[categorical_cols] = cat_imputer.fit_transform(df[categorical_cols]) # Detect and handle outliers using IQR method def remove_outliers(df, column): Q1 = df[column].quantile(0.25) Q3 = df[column].quantile(0.75) IQR = Q3 - Q1 lower_bound = Q1 - 1.5 * IQR upper_bound = Q3 + 1.5 * IQR return df[(df[column] >= lower_bound) & (df[column] <= upper_bound)] # Apply to numeric columns that need outlier detection df = remove_outliers(df, 'income') df = remove_outliers(df, 'age') print(f"Shape after cleaning: {df.shape}")
Best Practices
Always perform exploratory data analysis before preprocessing
Create preprocessing pipelines for reproducibility
Handle data leakage carefully when applying transformations
Scale features based on algorithm requirements
Document all preprocessing steps thoroughly
Test preprocessing impact on model performance
Process new data with the exact same pipeline
Balance preprocessing complexity against model needs
Continue Your Learning Journey
Ready to apply these preprocessing techniques? Check out these related tutorials to enhance your data science skills.