AI Agent Development
Autonomous AI
Build and deploy autonomous AI agents capable of reasoning, planning, and executing complex tasks
90 mins
Overview
- •Understanding autonomous AI agent architectures
- •Implementing reasoning and planning capabilities
- •Building agents with tool-using abilities
- •Multi-agent communication and coordination
- •Strategy for reliable agent evaluation
- •Deployment and monitoring of AI agents in production
Implementation Scenarios
Agent Architecture Design
FoundationDesign the core architecture for autonomous AI agents
Implementation Steps
- Understanding the ReAct (Reasoning + Acting) pattern
- Implementing prompt engineering for agent instructions
- Setting up memory systems for context retention
- Defining agent capabilities and limitations
- Creating personality and communication style
- Building safeguards and fallback mechanisms
Code Example
# Example code for implementing a ReAct agent architecture
from langchain_core.prompts import ChatPromptTemplate, MessagesPlaceholder
from langchain_openai import ChatOpenAI
from langchain.agents import AgentExecutor, create_react_agent
from langchain_core.tools import Tool
# Define the agent's system prompt with the ReAct pattern
SYSTEM_PROMPT = """You are an autonomous AI assistant tasked with helping users solve problems.
For each user request, follow these steps:
1. Thought: Think about what needs to be done to complete the task.
2. Action: Select a tool to use from the available tools.
3. Action Input: Provide the input for the tool.
4. Observation: After receiving the tool's output, analyze the information.
5. Thought: Reason about the observation and what to do next.
6. Action or Response: Either use another tool or respond to the user."""
# Define the agent's prompt template
prompt = ChatPromptTemplate.from_messages([
("system", SYSTEM_PROMPT),
MessagesPlaceholder(variable_name="chat_history"),
("human", "{input}"),
MessagesPlaceholder(variable_name="agent_scratchpad"),
])
# Create example tools
def calculator(expression: str) -> str:
"""Evaluate a mathematical expression."""
try:
return str(eval(expression))
except Exception as e:
return f"Error: {str(e)}"
def search_web(query: str) -> str:
"""Search the web for information."""
return f"Found results for: {query}. [Simulated search results]"
# Define the agent's tools
tools = [
Tool(name="Calculator", func=calculator, description="For mathematical calculations"),
Tool(name="Search", func=search_web, description="Search the web for information")
]
# Initialize LLM and agent
llm = ChatOpenAI(temperature=0, model="gpt-4o")
agent = create_react_agent(llm, tools, prompt)
agent_executor = AgentExecutor(agent=agent, tools=tools, verbose=True)
Tools & Libraries
LangChainOpenAI GPT-4ReAct PatternLLM Agents
Instructor
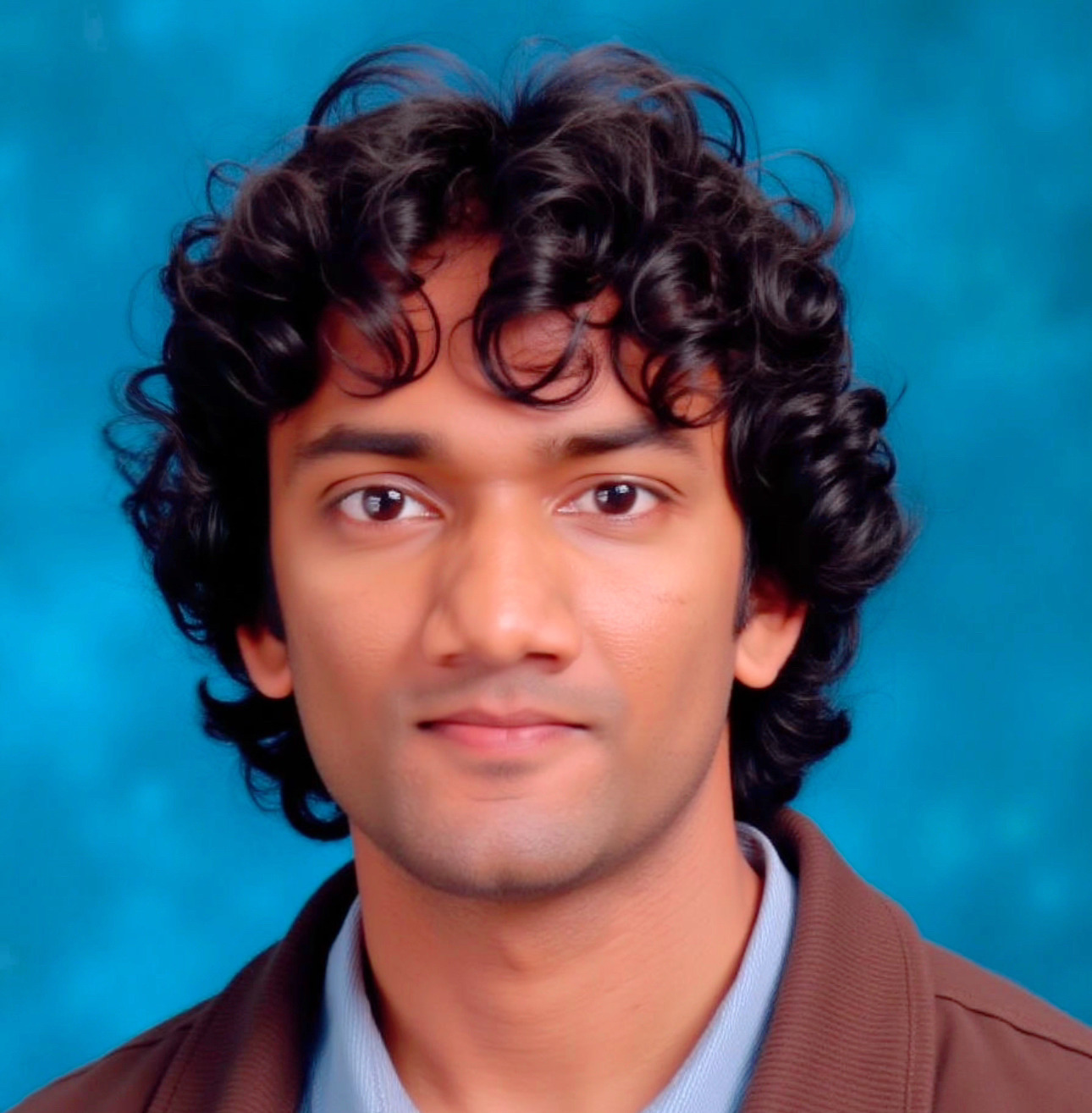
Nim Hewage
Co-founder & AI Strategy Consultant
Over 13 years of experience implementing AI solutions across Global Fortune 500 companies and startups. Specializes in enterprise-scale AI transformation, MLOps architecture, and AI governance frameworks.
Tutorial Materials
Additional Learning Resources
LangChain Agent Documentation
Comprehensive guide to implementing agents with LangChain
View documentation →